There are over 1.1 billion websites in the world, and most of them share one thing in common — they’re interactive. Users can submit forms, play games, navigate menus, move between pages, stream videos, and much more. Have you ever wondered what makes it all possible?
The answer often lies in JavaScript, one of the most widely used programming languages in the world. JavaScript powers almost 99 percent of websites globally and serves as the foundation for the biggest platforms, including Google, Meta, LinkedIn, and YouTube.
But JavaScript's versatility doesn’t stop at the browser — it has evolved into a full stack solution. This means developers can use it for both front-end and back-end development, creating seamless and unified applications.
In this article, we’ll break down what full stack JavaScript development is, how it works, the technologies involved, and why it’s become a go-to choice for modern software engineers. Let’s dive in!
What is JavaScript?
JavaScript is a high-level programming language used to build websites and web applications. Alongside HTML, which structures web pages, and CSS, which styles them, JavaScript plays a vital role in how we interact with the Internet.
Though it was created to add interactive features like animations, real-time updates, and form validations on the client side, JavaScript has also become a powerful tool on the server side making it a versatile and essential technology for modern web applications.
Origins and history of JavaScript
JavaScript — initially named Mocha, then LiveScript — was created in 1995 by Brendan Eich while working at Netscape Communications. It was eventually called JavaScript to capitalize on the growing popularity of Sun Microsystems' Java language.
Since its release, JavaScript has undergone several significant updates, transforming from a basic scripting tool for web pages to a robust programming language. These updates are defined by the ECMAScript standard (ECMA-262). The major versions of JavaScript include:
- ECMAScript 1 (1997) formalizing JavaScript's syntax and basic capabilities;
- ECMAScript 3 (1999) introducing regular expressions, try/catch statements for error handling, and string manipulation methods;
- ECMAScript 5 (2009) laying the groundwork for modern JavaScript development with strict mode, JSON support, and new array methods;
- ECMAScript 6/ECMAScript 2015 modernizing the language with improvements like let/const, arrow functions, promises, classes, modules, and template literals;
Since 2016, all versions of ECMAScript have been named by their release year, with the latest version being ECMAScript 2024. Improvements that have come in these years include features like nullish coalescing (??), optional chaining (?.), logical assignment operators (||= and &&=), top-level await, and array grouping methods like groupBy().
How does JavaScript work on a web page?
When a user enters a URL or clicks on a link, the server sends the HTML, CSS, and JavaScript code needed to render the content. Then, the browser parses the HTML file to create a Document Object Model (DOM) tree, which represents the page’s structure as a tree-like hierarchy of objects.
After the DOM is built, the next stage is rendering the page for the user to see. Two primary rendering methods available are client-side rendering (CSR) and server-side rendering (SSR). With CSR, the browser’s JS engine (like Google Chrome's V8 or Mozilla's SpiderMonkey) executes any JavaScript code it encounters directly on the user’s device. In contrast, SSR processes JavaScript on the server. Frameworks like Next.js and Nuxt.js enable the server to render a fully prepared HTML page before sending it to the browser.
Once the page is fully loaded, the browser continues monitoring user interactions in real time through event listeners that trigger specific functions when actions like clicks, scrolls, and keystrokes occur. For example, JavaScript might validate form inputs when a user submits a form or fetch more blog posts from a server as someone scrolls down a page.
What is JavaScript used for besides front-end development?
While JavaScript is heavily used in front-end web development, it is a versatile programming language that has other use cases.
Back-end development. JavaScript powers back-end development through runtime environments like Node.js and frameworks like Express.js, which allows developers to build APIs, write server-side code, and handle user requests to databases. Companies like Netflix, Twitter, and LinkedIn use these tools to build their back-end infrastructure.
Mobile app development. JavaScript has extended its reach to mobile app development with React Native and Ionic. They allow engineers to design hybrid apps that run on multiple platforms using a single JavaScript codebase. Meta and Shopify use React Native to build their mobile apps, while Airbus and Southwest Airlines rely on Ionic.
Machine learning. While not traditionally associated with machine learning, JavaScript has made it into this field through libraries like TensorFlow.js, ml5.js, and Brain.js. Developers can use them to create and train models directly in the browser or on the server. The ability to use machine learning in web applications and perform tasks like image recognition, natural language processing, and predictive analytics, creates room for new possibilities.
Game development. JavaScript powers game development with tools like Phaser, Babylon.js, and Three.js, which support both 2D and 3D game creation. Phaser is widely used for creating browser-based 2D games with engaging animations and interactivity, while Three.js and Babylon.js leverage WebGL to build immersive 3D environments directly in the browser. The accessibility of JavaScript makes it easy for aspiring game developers to create and share their games online.
Key JavaScript concepts and characteristics
Let’s explore the defining characteristics, concepts, and features of JavaScript that make it a highly favored programming language.
Dynamically-typed language
JavaScript is a dynamically typed language, meaning developers don’t need to declare variable types explicitly. Instead, the type is determined at runtime and can be changed. Consider the following example, where the variable message starts as a string type but is later reassigned as an integer.
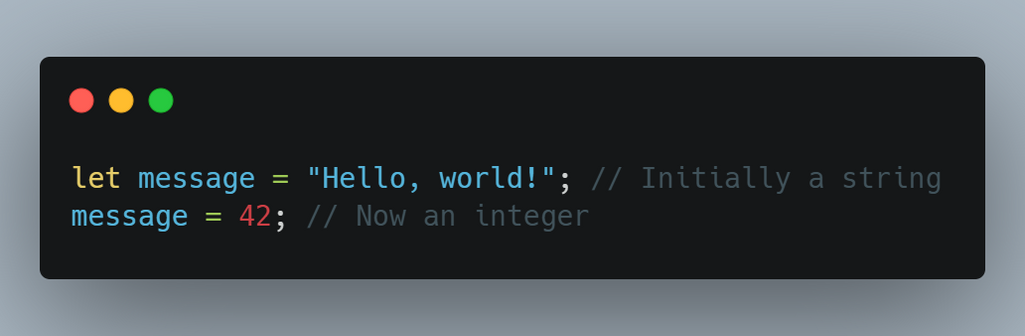
While the behavior, where variables can transition between numbers, strings, objects, and other data types without explicit type declarations, speeds up development, it can also lead to type-related errors if not managed carefully. Because of this, developers often use TypeScript to add static typing to JavaScript projects.
Single-threaded language
JavaScript is a single-threaded programming language, meaning it can only execute one task at a time on the main thread, which is responsible for running code and handling user interactions. This means the main thread can be blocked when performing time-consuming tasks like complex HTTP requests, large computations, or heavy DOM manipulations.
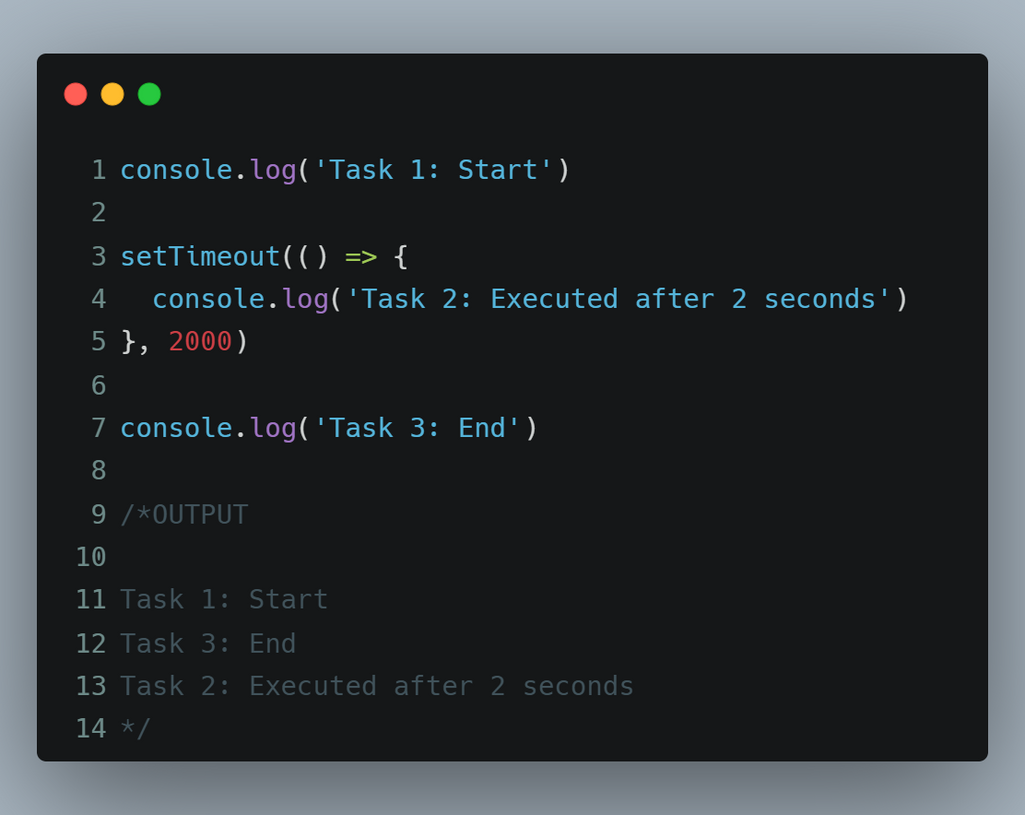
JavaScript’s single-threaded execution can lead to performance issues like a sluggish user interface, as the UI becomes unresponsive during these operations. To address this, JavaScript uses asynchronous programming techniques like Promises, Web Workers, and async/await to allow other tasks to run in parallel, which prevents the main thread from being blocked.
First-class functions
JavaScript treats functions as first-class citizens, meaning they can be assigned to variables, passed as arguments to other functions, returned from functions, and stored in data structures like arrays and objects.
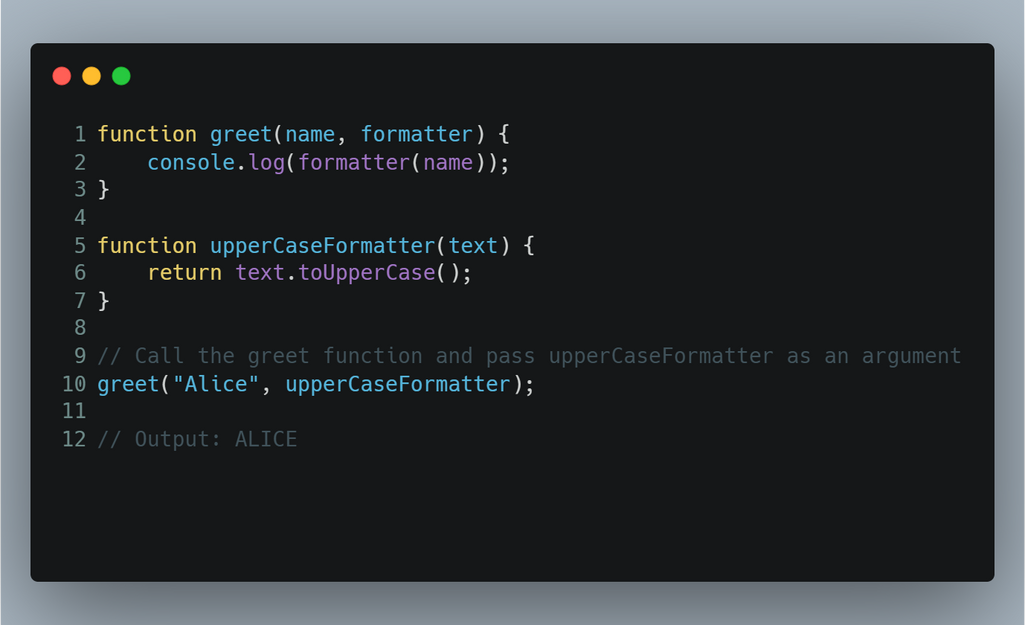
A JavaScript function greet() receives another function, upperCaseFormatter(), as one of its arguments
This feature powers built-in, higher-order functions like .map() and .filter() and allows for programming patterns like higher-order functions and callbacks.
Event-driven programming
JavaScript's event-driven model is an essential feature that allows developers to create interactive web applications that respond to user actions like clicks, keyboard inputs, or mouse movements through event listeners.
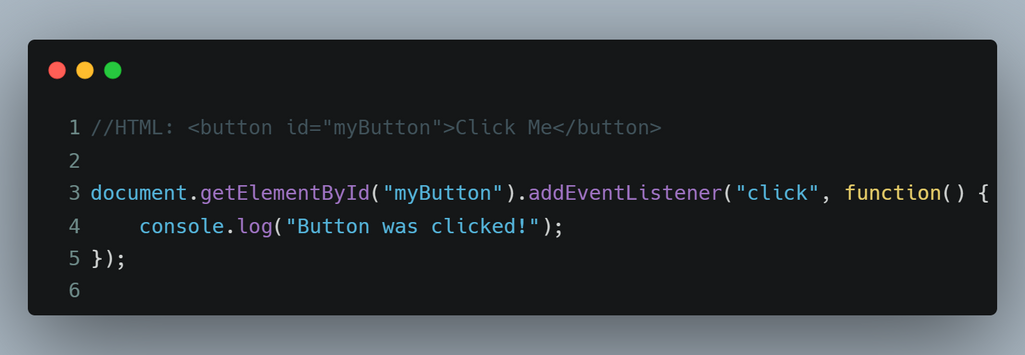
By attaching event listeners to HTML elements, developers can execute specific functions when events occur. This paradigm is essential for building responsive user interfaces where actions trigger immediate feedback — like displaying alerts or updating content dynamically — creating a seamless user experience.
While not a programming language, WebSockets are a communication protocols that utilizes event-driven architecture for real-time communication. Learn about it in our dedicated article.
The Document Object Model (DOM)
The DOM is a tree-like representation of an HTML document that JavaScript can interact with to manipulate web pages by modifying elements, adding events, or changing styles in real-time.
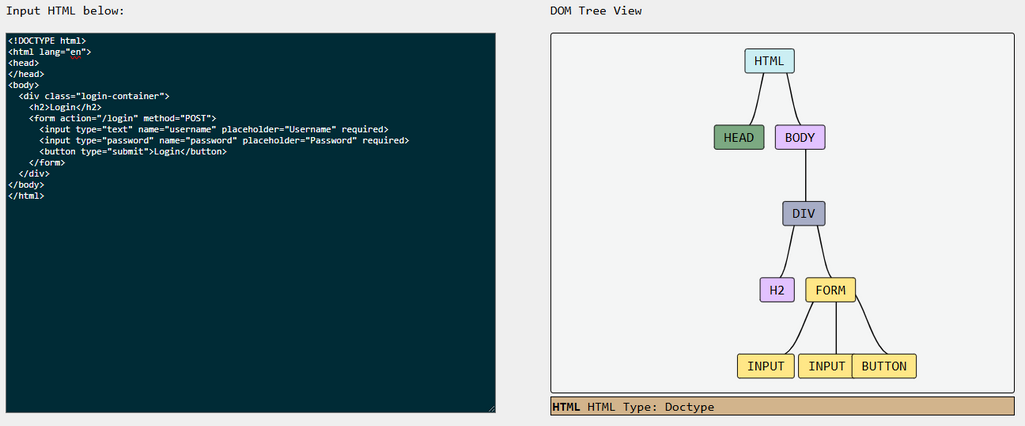
JavaScript provides multiple ways for developers to access, modify, and update the DOM. These include some of the following methods:
- Direct element selection: Using
document.getElementById()
to access elements by their ID ordocument.getElementsByClassName()
to access elements based on their CSS classes; - CSS selectors: Using
document.querySelector()
ordocument.querySelectorAll()
to target elements based on CSS selectors; - DOM traversal methods: Accessing parent, child, or sibling elements using properties like
parentNode
,children
, ornextElementSibling
; and - Event listeners: Capturing user interactions with the
addEventListener()
to dynamically modify the DOM.
An example of JavaScript DOM manipulation in action is the code snippet below, which performs some basic form validation to ensure no field is blank, and conditionally adjusts the text and color of the button based on the form’s state.
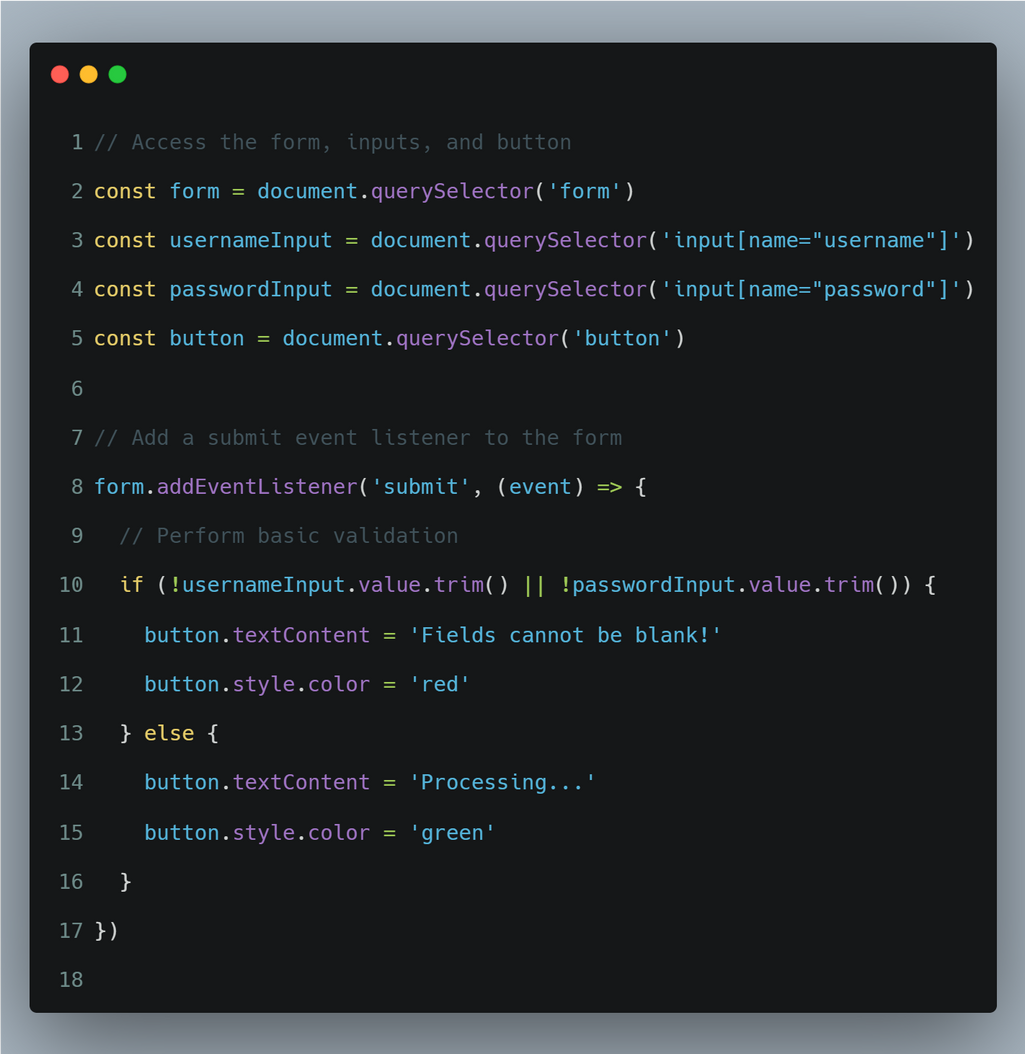
Explore the MDN docs to learn more about the DOM in detail, including the different DOM data types, events, and methods for accessing its elements.
What is a full stack JavaScript developer?
A full stack JavaScript developer is a software engineer skilled in using JavaScript and its ecosystem of tools to build both the front-end and back-end of an application. By using JavaScript technologies across the entire development process, these engineers create seamless and efficient web or mobile applications.
To succeed as full stack JS developers, professionals need expertise in the core JS language, front-end frameworks like React and Vue.js for creating dynamic user interfaces, and back-end tools like Node.js and Express.js for handling server-side operations.
Full stack JS developers have been highly sought after by startups and enterprises alike because their expertise reduces the need for separate front-end and back-end specialists. Instead, all layers of an application can be written in one language and by the same person.
Full stack JavaScript web development explained
Different combinations of JavaScript-based tools and frameworks are often referred to as stacks. The most popular of them are
- MEAN stack — MongoDB+Express+Angular+Node.js;
- MERN stack — MongoDB+Express+React+Node.js; and
- MEVN stack — MongoDB+Express+Vue.js+Node.js.
As you can see, they only differ by a front-end layer. Below, we examine how this component impacts the entire stack.
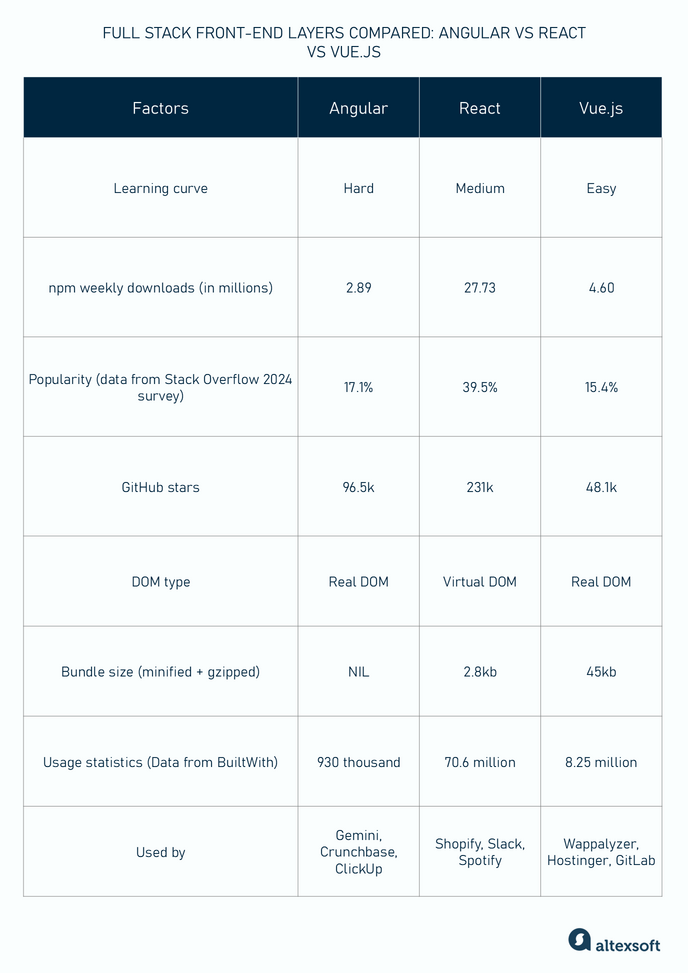
MEAN stack
When talking about full stack JavaScript, the first thing that comes to mind is the MEAN stack. It’s an open-source technology bundle that includes MongoDB, Express, Angular, and Node.js. Big companies like Google, Accenture, Sisense, and PayPal are embracing the MEAN approach. Let’s briefly discuss this stack’s components.
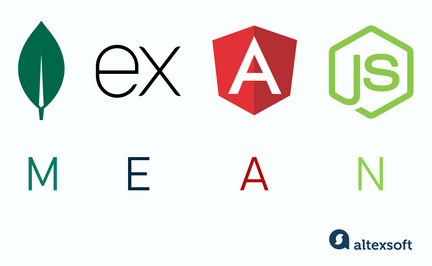
MongoDB is a NoSQL database that stores data in extended JSON (BSON) format. It offers schemaless flexibility and a horizontal scale-out architecture, making it ideal for handling big data.
Express.js is a back-end web application framework that’s built on Node.js and manages the workflow between the client side and the data model. It is used to create APIs, manage HTTP requests, and handle routing in web apps.
Angular is a popular front-end framework for creating dynamic user interfaces. It allows applications to interact with users without page reloads, improving performance and reducing bandwidth usage. For more information, check out our video on YouTube.
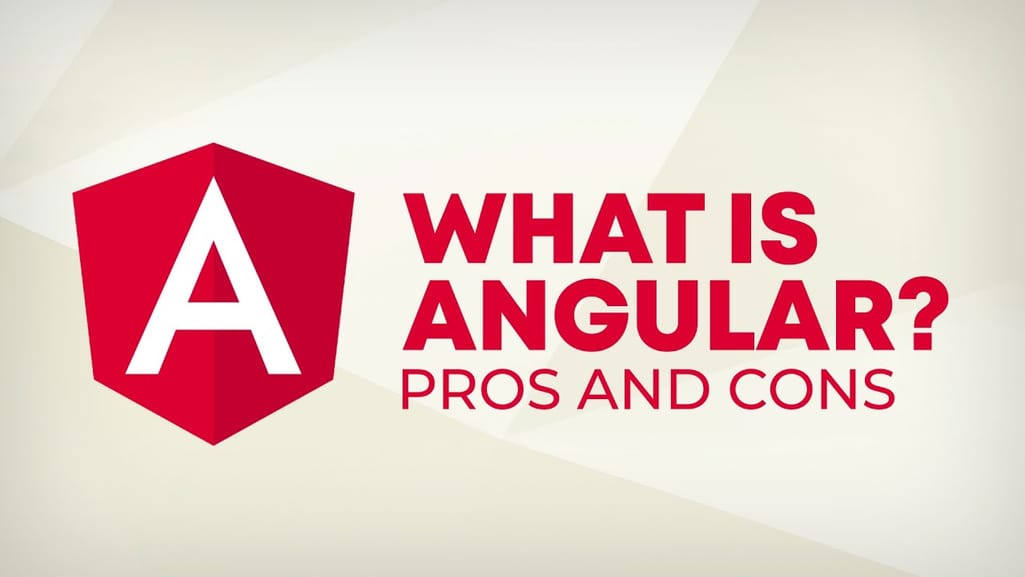
Node.js is a back-end JavaScript runtime environment that allows the JavaScript code to be executed outside the browser. It’s fast (running on the powerful V8 engine), lightweight, extremely efficient, and suitable for building data-intensive, real-time apps, so it’s no surprise it tops the list of most preferred frameworks.
MERN stack
MERN is very similar to MEAN with one exception — MERN relies on React — a front-end, open source library for building dynamic UI — instead of Angular.
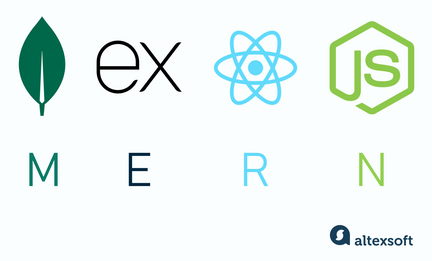
The pros and cons of the MERN stack vs MEAN stack are solely related to the difference between React and Angular. In short, React has an easier learning curve, ensures better performance due to its virtual DOM implementation, and provides bigger flexibility in tool usage. However, the latter might be considered a drawback since Angular is a complete framework and has everything you need to start developing right out of the box, while with React, you’ll have to find and choose additional libraries yourself.
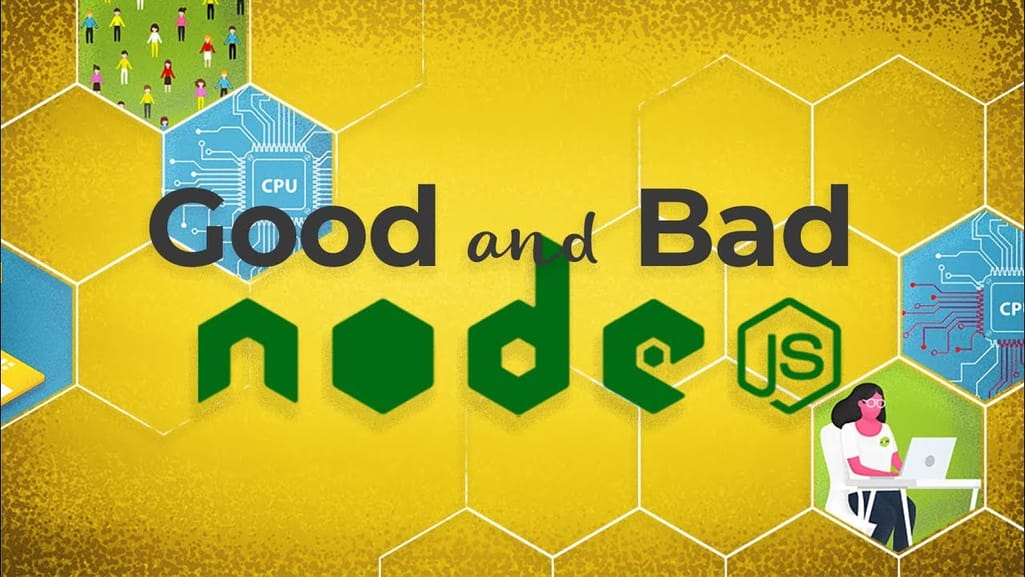
MEVN stack
Another member of the full stack JS family worth mentioning is MEVN — with Vue.js being the alternative framework for front-end development. Evan You, the creator of Vue.js, borrowed inspiration from the good parts of React and Angular, making Vue.js a good middle ground between both technologies. Vue.js is lightweight, flexible, and easy to learn.
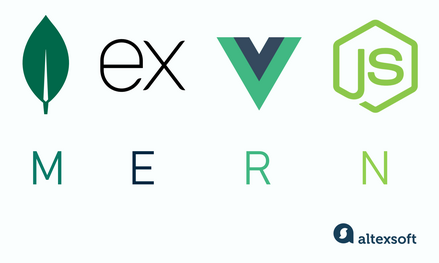
As of today, Vue.js already has 48k stars on GitHub, compared to React’s 230k and Angular’s 96.5k. The stack you use depends on factors like team expertise, project requirements, community support, and development timeline.
The pros of full stack JavaScript development
The fact that small-scale to large-scale companies like Groupon, Airbnb, Netflix, Medium, and PayPal adopt the full-stack JavaScript approach to build their products speaks for itself. This is mostly due to the number of benefits full-stack programming offers.
Common language, better team efficiency with fewer resources
Having all parts of your web application written in JavaScript allows for a better understanding of the source code within the team. Therefore, there is no such gap between front and back-end engineering that occurs when two teams are working separately using different technologies.
Moreover, you can now work with only one team instead of two for the back and front end, which should significantly reduce the cost and effort of finding and retaining the right talent. Such a cross-functional team is a great asset when following Agile methodologies.
Extensive code reuse
With full-stack JavaScript, you save time through code reuse and sharing. Following the "don't repeat yourself" (DRY) principle, you might be able to reduce the effort by reusing the parts of the code (or shared libraries, templates, and models) on both the back and front end that are very close in terms of logic and implementation.
In other words, you don't need to think about the JavaScript utility equivalents in Python or Ruby. You just use the same utility on the server and in the browser. Reducing the number of lines of code by up to 40 percent is also a valuable capability when refactoring and maintaining the source code.
High performance and speed
Node.js uses an event-driven, non-blocking IO model that makes it lightweight and fast as compared to other commonly used back-end technologies. To prove this, PayPal published a comprehensive report on the results they saw when migrating from Java to full-stack JavaScript.
The company was able to make the development almost 2 times faster while reducing the engineering personnel involved. Moreover, they have seen a dramatic improvement in performance, doubling the number of requests completed per second and decreasing the average response time by 35 percent for the same page. This means that the pages are served 200ms faster, which is definitely a noteworthy result.
Large and active community
Backed by giants like Facebook and Google, JavaScript has a powerful and fast-growing community. There are around 1.2 million repositories on GitHub and over 2.5 million JavaScript-related questions on Stack Overflow. SlashData also estimates that JavaScript’s community is 25.2 million members strong, the largest for any programming language.
Thanks to its large community, JavaScript enjoys a wealth of resources, solutions, and tools and is frequently updated and improved. Employers also benefit from the vast talent pool of JavaScript developers, making it easier to hire skilled professionals.
Free, open-source toolset
Most of the full-stack JavaScript development tools are free or open-source projects. This means you don't need to bear additional expenses for costly licenses or subscriptions. The open-source tools are updated regularly and evolve fast due to active community contributions. Instead of relying on a fixed set of technologies, you may use any of the numerous packages hosted by npm, the largest JavaScript module registry in the world.
Extensive documentation
Unlike some programming languages maintained by single entities or organizations, JavaScript is governed by the ECMAScript standard, which specifies how the language should work. As a result, there is no "official" JavaScript documentation.
Instead, the MDN Web Docs has become the most widely recognized and authoritative resource for JavaScript developers. MDN provides detailed explanations, examples, and references for every aspect of JavaScript, making it the go-to standard for developers worldwide.
Other resources like DevDocs also offer easily navigable and well-organized JavaScript references. DevDocs combines documentation from multiple sources into a single interface, making it easy for developers to access relevant information.
Ease of learning
Unlike other languages that require complex setups or additional installations, JS eliminates technical barriers by being immediately accessible to learners. All you need to get started is a web browser. Note that while the browser’s console is suitable for learning the basics, a code editor will be needed for more complex projects.
Another reason people often choose JavaScript as their first programming language is the ability to see immediate results. Since it’s primarily used for web-based projects, developers can directly interact with their code and see the outcomes in real time on the browser. This interactive experience motivates learners and encourages them to keep learning.
The cons of the full stack JavaScript approach
No technology is perfect. Despite all the benefits JavaScript offers, there are always some drawbacks to be aware of.
No type safety
While the flexibility of JavaScript’s dynamic nature can make coding faster, it also increases the risk of unexpected errors. For example, a variable initially assigned a number can later hold a string, leading to issues that are hard to debug.
Because there is no type enforcement, developers may accidentally introduce bugs that only surface at runtime.
While modern tools like TypeScript — which requires additional configuration and learning — address this challenge by adding optional static typing, JavaScript itself does not inherently support type safety, leading to weaker error handling in projects. As applications grow in complexity, debugging errors caused by type mismatches or implicit type coercion can become a significant pain point.
Security risks
JavaScript’s wide adoption and lack of built-in robust security mechanisms make it a prime target for security vulnerabilities. A major security issue is cross-site scripting (XSS), where malicious scripts are injected into web pages to steal user data or manipulate content. Another concern is code exposure, as client-side JavaScript code runs on the browser. Anyone can view a website's JavaScript code using browser developer tools, potentially exposing sensitive information or application logic.
No built-in cross-browser compatibility
Although JavaScript runs on all modern browsers, it is not inherently cross-browser-compatible. Different browsers interpret JavaScript differently, leading to inconsistent behavior or errors. For example, older versions of Internet Explorer often lack support for newer ECMAScript (ES) features, forcing developers to use polyfills (scripts that replicate missing features) or transpile their code using tools like Babel.
Testing and ensuring compatibility across multiple browsers is time-consuming and adds to the development cost. While modern JavaScript standards have improved consistency, developers must still ensure their code works seamlessly on all target browsers.
Insufficiency with computation-heavy back-end
When it comes to heavy computation and data processing on the server side, Node.js is not the best option. There are lots of far better technologies to handle machine learning and other heavy mathematical calculations.
Having a single CPU core and only one thread that processes one request at a time, it might be easily blocked by a single computationally intensive task. While the thread is busy processing the numbers, your application won’t be able to work with other requests, which might result in serious delays.
Yet, as we mentioned before, there are ways to overcome this limitation. By simply creating child processes or breaking complex tasks into smaller independent microservices that use more suitable technologies and communicate with your back end, you can handle complex computational tasks in Node.js.
Jack of all trades, master of none?
It is a common belief that a developer can truly master only one area of knowledge. With every other skill gained, the quality of his/her expertise could decline. While the syntax and grammar of JavaScript are mostly the same on the client and server side, there are still many details to consider.
Aside from being proficient in front end development, full stack JavaScript developers need to have an expertise in back end programming, such as HTTP protocol, asynchronous I/O, data storage fundamentals, cookies, etc. That is why some say that there are really no full stack engineers: Every one of them is either front or back end oriented. However, we have all the reasons to disagree based on our own experience and strong JavaScript skills.
Drawbacks of every separate tool in the stack combined
As any technology stack, MEAN/MERN/MEVN combines the weak sides of all 4 of its elements. Most of them are minor technical limitations, which appear under certain circumstances. However, in order to use the stack, it’s important to realize possible bottlenecks of every tool and adjust your development strategy accordingly.
Comparing JavaScript with other programming languages
While JavaScript is a most significant pillar of web development, it's not the only option for developers. Let’s compare it with others.
JavaScript vs Java
If developers had a dollar for every time someone asked if JavaScript and Java are the same thing, they’d probably be billionaires. Despite their similar names, they’re as related as a car is to a carpet. As mentioned earlier, JavaScript was intentionally branded that way to ride on Java’s popularity at the time.
In terms of differences, JavaScript is a dynamically-typed scripting language for making websites interactive while Java is a statically-typed language for building large-scale back-end applications, enterprise software, and Android development. Also, where JavaScript is an interpreted language that’s executed directly in a browser without a compilation step, Java code is compiled by the Java compiler (javac) and executed by the Java Virtual Machine (JVM).
JavaScript vs TypeScript
TypeScript, introduced by Microsoft in 2012, is a superset of JavaScript that was designed to address JS’s lack of strong typing. It adds static types which helps developers catch errors during development rather than at runtime. TypeScript also supports modern JavaScript features and compiles to plain JavaScript for compatibility.
TypeScript’s adoption has surged over the years. GitHub’s State of Open Source shows that it’s the second fastest growing programming language and the 3rd most popular language across OSS projects. While JavaScript is still leading in levels of usage, there’s a growing trend of teams starting their projects with or migrating their existing codebases to TypeScript, like Stripe, Etsy, and Telerik did.
JavaScript vs Python
Technically speaking, comparing JavaScript and Python is like comparing Messi with Lebron James, two different players in two different sports. JavaScript dominates the web development space and helps with building interactive web applications, while Python is favored in fields like data science, data analysis, machine learning, and AI development.
They are both widely used languages, and no one leads in terms of popularity. While surveys by JetBrains and Stack Overflow peg JavaScript as the most popular language, GitHub shows Python overtook JavaScript as the most popular language due to the increased demand for AI-enabled applications.
JavaScript and Python both provide tools for back-end development. With JavaScript, popular tools include Node.js and Express.js, while Python supports back-end development through frameworks like Django and Flask.
JavaScript vs JQuery
jQuery is a JavaScript library that was built in 2006 to simplify JavaScript development and remains in use on over 75 percent of websites worldwide. However, jQuery’s adoption has declined over the years due to the rise of modern JavaScript frameworks like React, Vue.js, and Angular, which offer more powerful tools for building dynamic user interfaces.
Additionally, native JavaScript (ES6+) now incorporates many features that once made jQuery indispensable and has provided modern improvements like using the querySelector and querySelectorAll methods for DOM manipulation, addEventListener for event handling, the Fetch API for making HTTP requests, and Promises or async/await for handling asynchronous operations.
Getting started with JavaScript
If you’re interested in diving into full stack JavaScript development, here are some useful resources to help you master both front-end and back-end technologies within the JavaScript ecosystem.
Documentation. As we’ve learned, the MDN documentation is one of the most comprehensive guides for JavaScript. It covers everything from basic to advanced concepts and is ideal for beginners and experts. If you want to dive into the technical aspects, the official ECMAScript specification provides information about JavaScript standards. You can also explore the documentation of your chosen front-end and back-end tools to learn more about them.
GitHub repo. While there’s no JavaScript repository for exploring JavaScript’s codebase, the following GitHub repos will be helpful in your learning journey.
- javascript-algorithms: Contains JavaScript-based examples of many popular algorithms and data structures;
- 30-days-of-javascript: Describes major JavaScript challenges;
- clean-code-javascript: covers clean code concepts implemented in JavaScript; and
- awesome--javascript: aggregates JavaScript frameworks, libraries, and learning materials.
Also, check airbnb/javascript — Airbnb’s JavaScript style guide.
Community. Checkout JavaScript communities on Reddit, Stack Overflow. There’s a dedicated JavaScript Reddit community tagged Learn JavaScript that’s strictly for asking questions, and there’s the full stack development subreddit.. You can also explore the freeCodeCamp forum.
Online courses. The JavaScript community is spoiled with choices when it comes to online courses. Some great ones are the MDN learning area, 30 seconds of code, Traversy Media’s YouTube crash course, and Net Ninja’s JavaScript tutorial for beginners. There’s also The Odin Project’s and Full stack open’s courses, which cover full stack development.
Books. If you prefer reading, you’re not left out. There are a lot of helpful JavaScript books, including Eloquent JavaScript, You Don’t Know JS, Full Stack JavaScript Development With MEAN, and Speaking JavaScript. Also, subscribe to the JavaScript Weekly newsletter to stay informed on the latest updates and open-source projects in the JavaScript ecosystem.
This post is a part of our “The Good and the Bad” series. For more information about the pros and cons of the most popular technologies, see the other articles from the series: