Apps that deliver messages the moment you hit the send button and update stock prices in the blink of an eye are now commonplace. The instantaneous data delivery we’ve grown accustomed to is powered by WebSockets, which enable instant interactions between systems. In this article, we’ll break down how WebSockets work, why they’re essential for real-time applications, and how they compare to other communication methods.
What is a WebSocket?
WebSocket is a communication protocol that enables real-time, two-way interactions between a client (e.g., a browser) and a server. Unlike traditional HTTP requests, where the client asks the server for updates when needed, WebSockets let parties send data to each other at any time, similar to how people at both ends of a call can speak simultaneously. This is called full-duplex communication, and it makes WebSockets ideal for applications that require instant updates.
As far as web communication is concerned, there are two major models: request-response and event-driven. In the request-response model, the client initiates communication, and the server responds accordingly (e.g., standard HTTP). In contrast, event-driven communication is characterized by systems where events trigger data flows. WebSockets follow the event-driven approach, enabling data to flow freely between clients and servers without repeated requests.
What are WebSockets used for?
WebSockets fit any scenario where real-time communication is essential. Common use cases include the following.
Chat applications. Instant messaging platforms rely on WebSockets to send and receive messages in real time.
Trading platforms. Financial trading platforms use WebSockets to stream stock prices, cryptocurrency values, and other financial data. This is particularly crucial in high-frequency trading scenarios where even milliseconds of delay can impact trading outcomes.
Social media platforms. These apps use WebSockets to provide immediate updates to users' feeds, notifications, and interaction counters.
Online gaming. Online multiplayer games require constant synchronization of game state between players. They use WebSockets to instantly transmit player actions, position updates, and game events.
Collaboration tools. Apps like Google Docs use WebSockets to sync changes made by multiple users. For more context, read how AltexSoft built a conferencing app based on WebSocket communication.
These use cases highlight the indispensability of WebSockets across different industries that rely on real-time communication.
WebSocket in Python, Golang, and other languages
WebSocket servers and clients can be built in any server-side programming language using dedicated libraries that streamline development, for example
- websockets, Django Channels, Tornado, Python Socket.IO, and Flask-SocketIO for WebSocket implementation in Python and its frameworks;
- gorilla/websocket and go-socket.io for Google’s Golang (Go);
- laravel-websockets for Laravel, a popular PHP framework; and
- ws and uWebSockets.js for Node.js, a runtime environment to run JavaScript on the server side.
Also, there are library families like Socket.IO (we’ll talk about it later) and SockJS that provide packages for WebSocket server and client implementation across different languages.
How do WebSockets work?
WebSocket technology is built on two key components: the WebSocket API and the WebSocket Protocol. Let’s examine these elements in depth to better understand how WebSockets work.
WebSocket API
The WebSocket API provides a JavaScript interface for creating and managing WebSocket connections in web applications. It offers the following key methods and properties:
- WebSocket() constructor for creating new WebSocket connections;
- onopen event handler triggered when the connection is successfully established;
- onmessage event handler Invoked when a message is received from the server;
- onclose event handler fired when the connection is closed;
- onerror event handler called when an error occurs during communication;
- send() method that allows the client to send messages to the server; and
- close() method that closes the WebSocket connection.
The image below is an example of how the WebSocket API might be implemented:
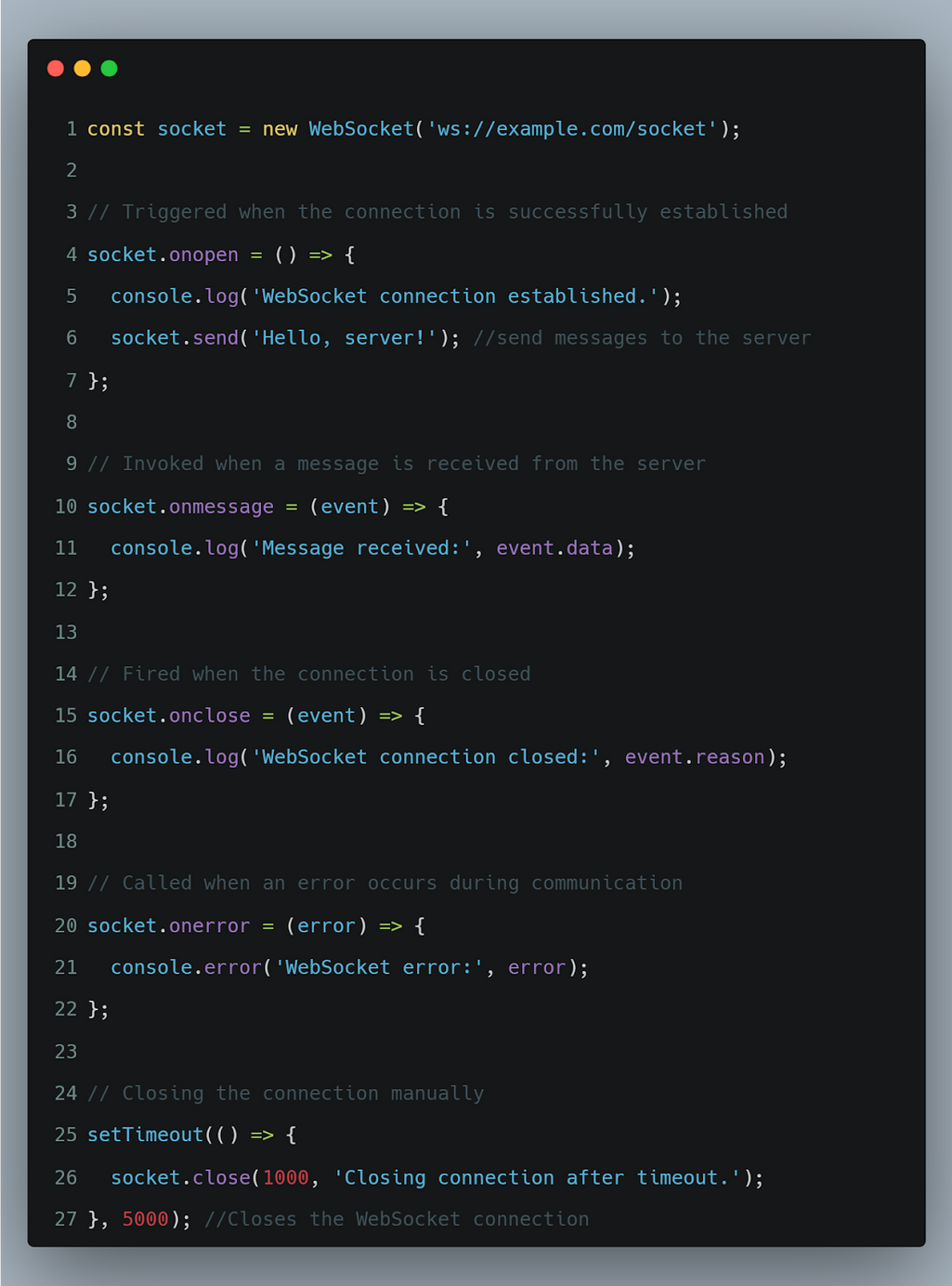
You can learn more about the WebSocket API and how to write a WebSocket client and server for communication via the API on the MDN documentation site.
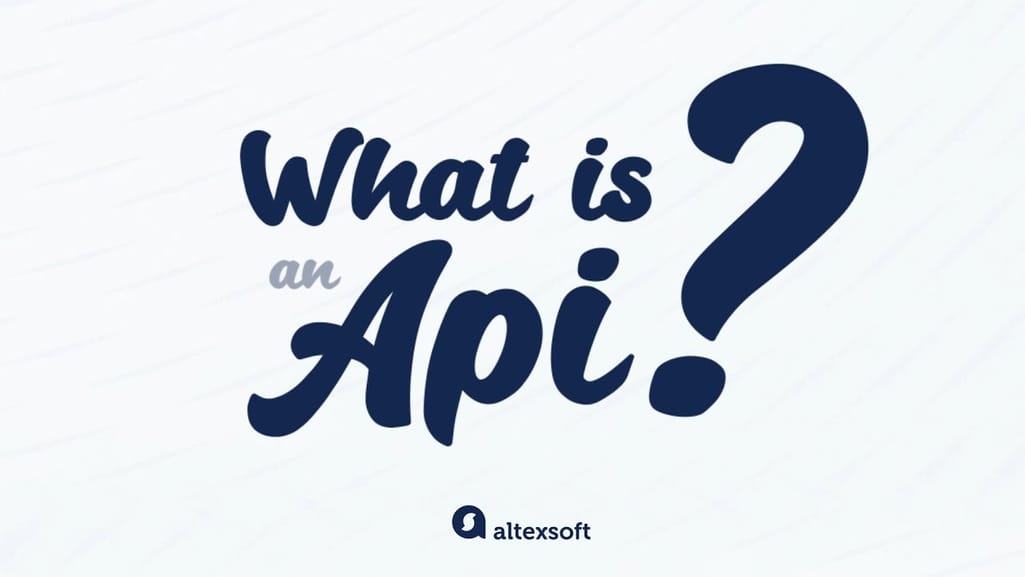
WebSocket protocol
The WebSocket Protocol defines the mechanics of WebSockets, which allow real-time data exchange between a client and a server over a Transmission Control Protocol (TCP), one of the main standards for Internet communications.
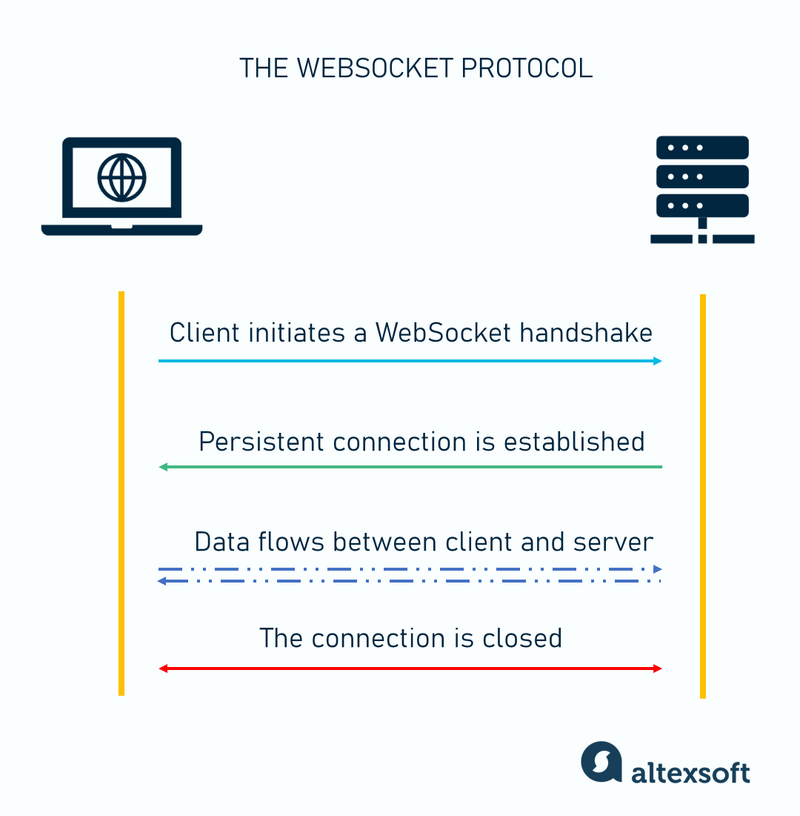
Here are the steps the WebSocket Protocol goes through to set up a connection.
Step 1: Handshaking. The process begins with a so-called opening handshake: The client sends an HTTP request to the server, asking to establish a WebSocket connection. If the server supports WebSockets, it responds with a confirmation and signals that the connection has been upgraded from HTTP to WebSocket.
Step 2: Establishing a persistent connection. Once the handshake is successful, the full-duplex WebSocket connection is established over TCP and stays open, allowing the client and server to send messages back and forth.
Step 3: Transferring data. Data now flows between the client and the server through data frames — the basic units of communication used to send data between the client and the server. The frames can carry text, binary data, or control messages. Both the client and server can send frames independently.
Step 4: Closing the connection. To terminate a WebSocket connection, either party can send a close frame, which includes a status code indicating the reason for closure. Upon receiving the close frame, the other party responds with an acknowledgment, and the connection is closed.
WebSockets vs other communication technologies
WebSockets are one of the many communication technologies for managing data exchange between clients and servers. Let’s explore how WebSockets compare to these alternatives.
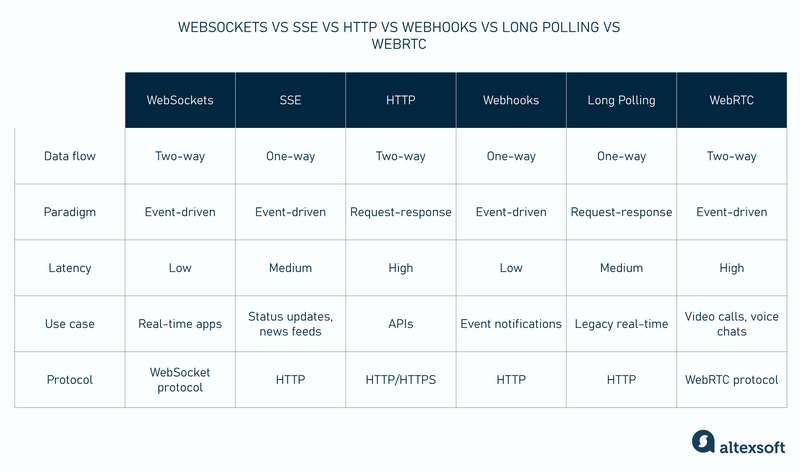
WebSockets vs SSE vs HTTP vs Webhooks vs Long polling vs WebRTC
WebSockets vs SSE
Unlike WebSockets that facilitate bidirectional data flow, server-sent events (SSE) are for unidirectional communication where only the server can push data to the client. This makes SSE suitable for scenarios like news feeds or status and score updates where the client doesn’t need to respond to the server or trigger events.
SSE is based on HTTP while WebSockets operate on a separate lightweight protocol that critically reduces latency. At the same time, SSE has a built-in reconnection mechanism that automatically fixes disruptions. With WebSockets, it’s up to a developer to introduce reconnecting capabilities. Overall, SSE is simpler to implement than WebSockets.
WebSockets vs HTTP
The HTTP protocol follows the request-response model, where the client initiates all communication by sending requests to the server, which responds with data. As we said earlier, HTTP communication has a high latency compared to WebSockets. It doesn’t support real-time interactions. Instead, it's well suited for delivering static content, responding to user actions, and performing CRUD operations via REST APIs.
WebSockets vs webhooks
Webhooks are user-defined HTTP callbacks that allow one application to send real-time data to another whenever specific events occur. Webhooks differ from other mechanisms as they primarily facilitate server-to-server communication.
Unlike WebSockets, webhooks can only send data from the source to the destination; there is no response from the receiver to the sender. Webhooks operate an event-driven model and are commonly used for third-party integrations (for example, with payment gateways), updating eCommerce websites, or triggering CI/CD pipelines.
WebSockets vs long polling
Long polling is one of the first attempts to simulate real-time communication over HTTP. In this approach, the client makes a request to the server, and the server keeps the connection open until new data is available. If the client has to send information back to the server, a separate HTTP request is created
Long polling is less efficient than WebSockets as it involves repeatedly establishing HTTP connections, adding to overhead and latency. Because of this, it is better suited for applications with low-frequency updates.
WebSockets vs WebRTC
Unlike WebSockets, which facilitate client-server communication, Web Real-Time Communication (WebRTC) is a peer-to-peer protocol designed for real-time audio, video, and data sharing between browsers. It is ideal for applications like video conferencing, voice calls, peer-to-peer file sharing, and streaming apps.
WebSocket tools for implementing real-time functionality in applications
While you can write WebSocket connections from scratch, you don’t need to, as there are WebSocket servers that simplify the process of setting up these communications.
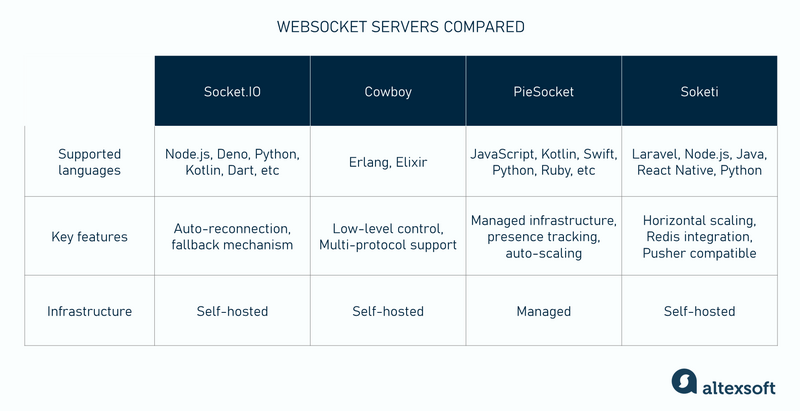
Socket.IO
Socket.IO is one of the most widely used WebSocket libraries for real-time communication in JavaScript applications. The latest version supports various client and server languages, including Node.js, Deno, Python, Kotlin, and Dart.
Socket.IO uses WebSocket as its primary protocol, but its fallback feature allows it to automatically switch to other protocols when WebSockets are unavailable. Socket.IO ensures a stable connection by automatically reconnecting the client to the server in case of disruptions.
As a self-hosted solution, Socket.IO gives developers complete control over their infrastructure.
Cowboy
Cowboy is an HTTP server that supports WebSockets and other HTTP-based protocols. It is built on Erlang's concurrency model and can handle multiple concurrent connections without sacrificing performance, making it ideal for building scalable real-time applications.
Cowboy handles the WebSocket protocol at a lower level than solutions like Socket.IO, giving developers more control over the connection lifecycle and message handling. Its multi-protocol support makes it an excellent choice for complex applications requiring various protocols.
Cowboy is a self-hosted solution that offers developers full control over their server setup.
PieSocket by PieHost
PieHost is a fully managed platform that provides WebSocket as a service via PieSocket, one of its offerings. PieSocket abstracts away much of the complexity of setting up a WebSocket server, making it an excellent option for developers who need a managed solution with minimal configuration. It supports various languages, including JavaScript, Kotlin, Swift, Python, and Ruby.
PieSocket provides features like built-in presence tracking for monitoring user activity in real-time and auto-scaling, which ensures that applications can handle sudden traffic spikes seamlessly.
As a managed service, PieSocket removes the need for server maintenance, enabling developers to focus solely on development.
Soketi
Soketi is a modern WebSocket server designed for high performance. It was originally written in C and has been ported to Node.js. This allows it to leverage Node.js features and optimizations, making it capable of handling large numbers of concurrent connections efficiently.
Soketi integrates with various languages — Laravel, Node.js, Java, React Native, Python, etc. — and comes with easy deployment options, including Docker support. It scales horizontally and uses Redis Pub/Sub to efficiently broadcast messages throughout multiple instances. Soketi is also Pusher-compatible, allowing developers to easily migrate existing applications or integrate with current setups.
As a self-hosted solution, Soketi offers flexible deployment options, including Docker.
WebSocket testing
An important aspect of WebSocket development is WebSocket API testing, which ensures that WebSocket API connections are fully reliable, performant, and secure. There are various types of tests you can perform, including
- functional testing to ensure that WebSocket endpoints and messages operate as expected;
- performance testing to evaluate the time taken to transmit and receive messages, the volume of messages that can be processed in a given time, and the system’s ability to handle thousands or millions of simultaneous connections;
- security testing to verify data encryption, validate access controls, and assess vulnerability to injection attacks, data leakage, and other common threats;
- load testing to check how the system behaves under heavy load; and
- integration testing to see how WebSockets interact with other components of the application, like APIs, databases, and front-end interfaces.
You can use a range of tools for WebSocket API testing. Solid options include Postman, Apache JMeter, and WebSocket King.
Only so much can be covered in a single article. Explore the following articles to learn more about WebSockets, APIs, and other technologies in the web services ecosystem: